今回は、AmazonAlexaやGoogleアシスタントなどに興味があって前からやってみたかったのですが、Pythonでマイクから入力した音声をテキストにおとしすることにトライしてみましたので、記事にします。
ライブラリのインストール
入力音声をテキスト化するためにまずはライブラリのインストールから行っていきます。
今回インストールするライブラリはSpeechRecognistionというものを使用します。
いつもどおりpipコマンドを使用してインストールしていきます。
コマンドプロンプトを起動し、以下の通り入力してください。
pip install SpeechRecognistion
以下のように、Successfully installed SpeechRecognition-XXXXと出ればインストール完了です。

オフライン環境でインストールしたい場合は以下から、whlファイルをダウンロードしてSpeechRecognitionの代わりにファイルを指定してインストールしてください。
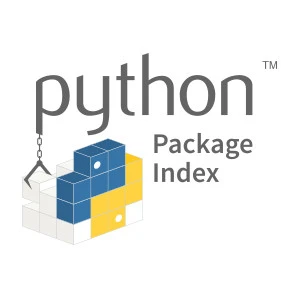
サンプルコード
では、まず、コード全体をどうぞ。
import sys
import os
import pyaudio
import wave
import time
import threading
from logging import NullHandler
import speech_recognition
record_filepath = "D:\\Pythonテスト\\SpeechRecognition\\rec.wav"
rectime = 5
wave_f = NullHandler
streamstop = False
def readaudio(filepath):
global wave_f
global streamstop
# Audio インスタンス取得
audio = pyaudio.PyAudio()
stream = audio.open( format = pyaudio.paInt16,
rate = 44100,
channels = 1,
input_device_index = 1,
input = True,
frames_per_buffer = 4096,
)
wave_f = wave.open(filepath, 'wb')
wave_f.setnchannels(1)
wave_f.setsampwidth(2)
wave_f.setframerate(44100 )
while True:
if streamstop == False:
data = stream.read(4096)
rec_exec(data)
else:
break
# 音声を読み出し
wave_f.close()
# Audio デバイスの解放
stream.stop_stream()
stream.close()
audio.terminate()
def main_func():
global streamstop
print(record_filepath)
thread1 = threading.Thread(target=readaudio, args=(record_filepath,))
thread1.start()
count = 0
while True:
try:
time.sleep(1)
count += 1
if count >= rectime:
streamstop = True
break
except KeyboardInterrupt:
streamstop = True
break
thread1.join()
# ここから認識
print("######Start Speech Recognition######")
sprec = speech_recognition.Recognizer() # インスタンスを生成
with speech_recognition.AudioFile(record_filepath) as sprec_file:
sprec_audio = sprec.record(sprec_file)
sprec_text = sprec.recognize_google(sprec_audio, language='ja-JP')
print(sprec_text)
print("######Finish Speech Recognition######")
os.remove(record_filepath)
def rec_exec(data):
global wave_f
#print(data)
wave_f.writeframes( (data) )
if __name__ == '__main__':
main_func()
プログラム全容
まずは、このコードで何をしているかを解説します。
今回使用するライブラリは、Wavファイルを渡す必要があるようでしたので、まず音声の録音を行います。
「D:\Pythonテスト\SpeechRecognition」というフォルダに、”rec.wav”というファイルに、rectime秒間(今回は5秒間)音声を録音します。
※何かキーを入力すると、5秒待たずに終了します。
続いて今回の本題となるSpeechRecognitionに録音したデータを引き渡しし、テキストに変換しています。
テキストを出力した後は作成した音声データを破棄して終了します。
プログラムの実行結果は以下の通りで、私がテストで話した内容がしっかりテキストとして出力されています。

続いてはプログラムの解説ですが、解説は次ページにて記載します。
コメント